Machine Learning With the Iris Dataset
Technology used: Python
My Contribution: Solo (Class Project and Tutorial)
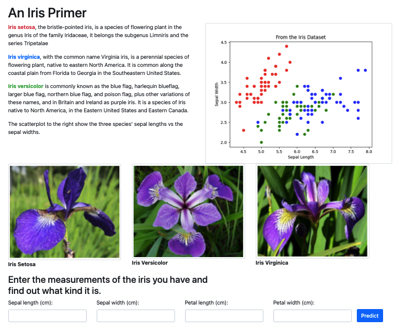
The class exercise was part of a multi week project building a machine learning application without using any python libraries.
The Tutorial is doing the same thing just with python libraries numpy, pandas, joblib, io and matplotlib. And using Flask, a user interface was quickly added.
What was challenging
For the class project, the challenge was understanding how the different functions fed into eachother, and what the individual function returned - in other words how the data flowed.
What I learned
Working on these projects showed me the mechanics of machine leaning becasue we either had to code functions ourselves or understand the flow in an instructor given function in order to make our own functions work.
””” Network class teaching the network “””
class FFBPNetwork:
# Use if user tries to add layer with less than one Neurode
class EmptyLayerException(Exception):
pass
# Use if our NNData object has no examples loaded.
class EmptySetException(Exception):
pass
def __init__(self, num_inputs, num_outputs):
self.layers = LayerList(num_inputs, num_outputs)
def add_hidden_layer(self, num_neurodes=5):
if num_neurodes < 1:
raise FFBPNetwork.EmptyLayerException
else:
self.layers.insert_hidden_layer(num_neurodes)
def remove_hidden_layer(self):
self.layers.current.rev_iterate().remove_hidden_layer()
def iterate(self):
return self.iterate()
def rev_iterate(self):
return self.rev_iterate()
def reset_cur(self):
return self.reset_cur()
def get_layer_info(self):
return self.layers.current.get_layer_info()
def train(self, data_set: NNData, epochs=1000, verbosity=0, order=NNData.Order.SEQUENTIAL):
if data_set == 0:
raise FFBPNetwork.EmptySetException
# rms_error = 0
running_error_total = 0
for epoch in range(epochs):
running_error_total = 0
# rms_error = 0
data_set.prime_data(order)
for one_sample_training_loop in range(data_set.get_number_samples(NNData.Set.TRAIN)):
x, y = data_set.get_one_item(NNData.Set.TRAIN)
for i in range(len(x)):
self.layers.get_input_nodes()[i].receive_input(None, x[i])
example_error_total = 0
for i in range(len(y)):
one_error = (self.layers.get_output_nodes()[i].value - y[i]) ** 2
example_error_total = example_error_total + one_error
running_error_total = running_error_total + math.sqrt(example_error_total)
for i in range(len(y)):
self.layers.get_output_nodes()[i].receive_back_input(None, y[i])
if verbosity > 1:
if epoch % 1000 == 0:
output_node_list = []
for n in self.layers.get_output_nodes():
output_node_list.append(n.value)
print("verbosity > 1", output_node_list, y)
if verbosity > 0:
if epoch % 100 == 0:
rms_error = math.sqrt(running_error_total / data_set.get_number_samples())
print("RMSE for epoch", epoch, ":", rms_error)
rms_error = math.sqrt(running_error_total / data_set.get_number_samples())
print("RMS Error for last epoch:", rms_error)
return rms_error
def test(self, data_set: NNData, order=NNData.Order.SEQUENTIAL):
running_error_total = 0
data_set.prime_data(order)
for one_test_loop in range(data_set.get_number_samples(NNData.Set.TEST)):
x, y = data_set.get_one_item(NNData.Set.TEST)
for i in range(len(x)):
self.layers.get_input_nodes()[i].receive_input(None, x[i])
predicted_value_list = []
for i in range(len(y)):
predicted_value_list.append(self.layers.get_output_nodes()[i].value)
example_error_total = 0
for i in range(len(y)):
one_error = (self.layers.get_output_nodes()[i].value - y[i]) ** 2
example_error_total = example_error_total + one_error
running_error_total = running_error_total + math.sqrt(example_error_total)
print(x, y, predicted_value_list)
# For easy copy and paste for graph
# print(x[0], y[0], predicted_value_list[0])
rms_error = math.sqrt(running_error_total / data_set.get_number_samples())
print("RMS Error for test data", rms_error)